SMPP Lua Service
Introduction
The SMPPLuaService is a service for initiating Lua scripts running within the LogicApp.
The SMPPLuaService receives an SMPP-S-REQUEST message message from an instance of the SMPPApp which is configured to receive SMPP requests from an external client.
The SMPPLuaService coordinates the loading of a Lua script. The script logic will perform any processing,
including access of any appropriate database, or external third-party components e.g. via REST, SOAP, or
any other protocol supported by the n2svcd
framework.
The SMPPLuaService will finally send an SMPP-S-RESPONSE message message back to the originating SMPPApp which will convey this as an SMPP protocol response back to the originating client.
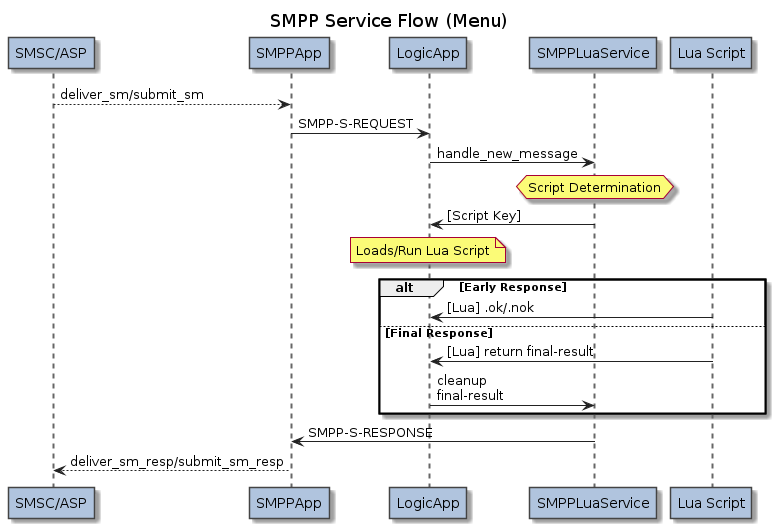
Configuring SMPPLuaService
The SMPPLuaService is configured within a LogicApp instance.
<?xml version="1.0" encoding="utf-8"?>
<n2svcd>
...
<applications>
...
<application name="Logic" module="LogicApp">
<include>
<lib>../apps/logic/lib</lib>
</include>
<parameters>
...
</parameters>
<config>
<services>
<service module="SMPPApp::SMPPLuaService" libs="../apps/smpp/lib" script_dir="/var/lib/n2svcd/logic/smpp">
<triggers>
<trigger pdu="deliver" source="641234567" destination="123" script_key="123"/>
<trigger pdu="submit" source_prefix="64" destination_prefix="123" script_key="123"/>
</triggers>
</service>
</services>
<agents>
...
</agents>
</config>
</application>
...
</application>
...
</n2svcd>
In addition to the Common LogicApp Service Configuration, note the following specific attribute notes, and service-specific attributes.
Under normal installation, the following service
attributes apply:
Parameter Name | Type | XML Type | Description |
---|---|---|---|
module
|
String | Attribute |
[Required] The module name containing the Lua Service code: SMPPApp::SMPPLuaService
|
libs
|
String | Element |
Location of the module for SMPPLuaService.(Default: ../apps/smpp/lib )
|
script_dir
|
String | Attribute | [Required] The directory containing scripts used by this service. |
.triggers
|
Array | Element |
Array of trigger elements specifying Lua scripts to run for SMPP Inbound Transactions.
|
.trigger
|
Object | Element | Provisions a Lua script to run for an SMPP Inbound Transaction for a destination/source party. |
Script Trigger Rules
Each SMPP trigger rule defines the name of a script which is ready to handle an SMPP Inbound Transaction.
Note that destination and source addresses may contain hex digits A-F, and the
matching is for destination_prefix
and source_prefix
is case-insensitive.
Each trigger
Object in the config
.triggers
Array is configured as follows.
Parameter Name | Type | XML Type | Description |
---|---|---|---|
destination
|
String | Attribute |
This trigger only applies for SMPP messages to this exact destination party. (Default = Trigger applies to all SMPP messages). |
destination_prefix
|
String | Attribute |
This trigger applies for only SMPP messages to destination parties starting with this prefix. (Default = Trigger applies to all SMPP messages). |
source
|
String | Attribute |
This trigger applies for only SMPP messages from this exact source party. (Default = Trigger applies to all SMPP messages). |
source_prefix
|
String | Attribute |
This trigger applies for only SMPP messages from source parties starting with this prefix. (Default = Trigger applies to all SMPP messages). |
script_key
|
String | Attribute |
The name for the Lua script to load (excluding the ".lua" or ".lc" suffix). The script should reside in the configured script_dir directory.
|
Script Selection (SMPP Transaction Request)
The Lua Script selection to execute for an SMPP message takes into consideration the source and destination addresses.
The destination address for matching is the SMPP parameter destination_addr
.
The source address for matching is the SMPP parameter source_addr
.
The SMPPLuaService will iterate the configured trigger
entries in the sequence given
and use the first trigger that matches the parameters of the received message.
Refer to the LogicApp configuration for more information on directories, library paths, and script caching parameters.
Script Global Variables
Scripts run with this service have access to the Common LUA Service Global Variables.
There are no service-specific global variables.
Script Entry Parameters (SMPP Request)
The Lua script defines the service processing steps, such as the following:
local n2svcd = require "n2.n2svcd"
local smpp_service = require "n2.n2svcd.smpp_service"
local args = ...
if (args.smpp.fields.short_message_text == 'Valid') then
return smpp_service.ESME_OK
else
smpp_service.nok (smpp_service.ESME_RUNKNOWNERR)
n2svcd.write_edr ('DECLINED', { BAD_CONTENT = smpp.fields.short_message_text })
return smpp_service.ESME_RUNKNOWNERR
end
The service script will be executed with an args
entry parameter contains the smpp
object
of the received SMPP-S-REQUEST message.
Script Return Parameters (SMPP Response)
The Lua script is responsible for determing the contents of the response SMPP deliver_sm_resp
or submit_sm_resp
(as appropriate to the received SMPP message) that will be sent back to the message source.
There are three mechanisms for determining the final success/reject result of message handling.
- Return
nil
to indicate success result without message ID returned. - Return a simple integer command status value, e.g. one of the
ESME_
constants defined in the service module. - Return a table containing
command_status
and optionalfields
sub-table withmessage_id
. - Use either the
nok
orok
method expressly, in which case the final script return value is ignored.
To summarise these in slightly more detail.
(1) Returning nil
at the end of the script indicates that the sm_deliver_resp
or sm_submit_resp
processing
response will contain command status (0 = ESME_OK) and the message ID is not present.
Example (returning a successful response using nil):
local n2svcd = require "n2.n2svcd"
local args = ...
return nil
(2) Returning a Lua Number result at the end of the script indicates that the sm_deliver_resp
or sm_submit_resp
processing response will contain command status with the indicated value, and the message ID is not present.
Example (returning an error response using Lua Number return):
local n2svcd = require "n2.n2svcd"
local smpp_service = require "n2.n2svcd.smpp_service"
local args = ...
return smpp_service.ESME_RINVDFTMSGID
(3) Returning Lua Table result at the end of the script, the table must be a response
object which has the
structure of the smpp
object of the SMPP-S-RESPONSE message.
Note that the Message ID field is only relevant for submit_sm_resp
.
Example (returning a success with message ID using Lua Table return):
local n2svcd = require "n2.n2svcd"
local args = ...
return { command_status = 0, message_id = 'ID7777' }
(4) Refer to the per-method documentation below.
The SMPPLuaService API
The SMPP Service API can be loaded as follows.
local smpp_service = require "n2.n2svcd.smpp_service"
It is not necessary to load the SMPP Service API if you are only using the simple response mechanism described above. It is only required if you wish to use any of the extended features described below, or any of the predefined error constants.
.response [Synchronous]
When a Lua script needs to perform extended processing, it may wish to send an early SMPP
response before the script completes. This can be done with the response
method on the
SMPP Service API.
This method will request the handling SMPPApp to send the appropriate submit_sm_resp
or
deliver_sm_resp
message back to the originating client.
The response
method takes a single response
parameter.
Parameter | Type | Description |
---|---|---|
response
|
Table |
A Lua Table with the same structure described under "Script Return Parameters", i.e.
which has the structure of the smpp object of the SMPP-S-RESPONSE message.
Note that the Message ID field is only relevant for `submit_sm_resp`.
|
After the response
method returns, the SMPP response has been handled, and the return
value of the service script is now ignored.
The response
method returns nil
.
[Fragment] Example Early Response success specifying message ID:
...
smpp_service.response ({ command_status = 0, message_id = assigned_id })
[post-processing logic after SMPP transaction is finished]
...
[Fragment] Example Early Response failure:
...
smpp_service.response ({ command_status = 0x44 })
[post-processing logic after SMPP transaction is finished]
...
.ok [Synchronous]
The ok
method is a short-cut for the early sending of a successful response,
i.e. one with command_status
= ESME_OK
= 0
.
The ok
method takes a single optional parameter.
Parameter | Type | Description |
---|---|---|
message_id
|
String | Optional message ID field to include in the response, for submit_sm handling only. |
The ok
method returns nil
.
[Fragment] Example Early Response success specifying message ID:
...
smpp_service.ok (assigned_id)
[post-processing logic after SMPP transaction is finished]
...
.nok [Synchronous]
The nok
method is a short-cut for the early sending of an unsuccessful response,
i.e. one with command_status
not equal to ESME_OK
= 0
.
The nok
method takes a single mandatory parameter.
Parameter | Type | Description |
---|---|---|
command_status
|
Integer | Mandatory non-zero command status. |
The nok
method returns nil
.
[Fragment] Example Early Response failure:
...
smpp_service.nok (0x44)
[post-processing logic after SMPP transaction is finished]
...
Constants
The following SMPP constants are defined on the returned smpp_service
object.
SMPP Command Status Constants
The following constants are the pre-defined, non-reserved SMPP 3.4 constant command status values.
-- SMPP Command Status Constants.
smpp_service.ESME_ROK = 0x00000000 -- No Error
smpp_service.ESME_RINVMSGLEN = 0x00000001 -- Message Length is invalid
smpp_service.ESME_RINVCMDLEN = 0x00000002 -- Command Length is invalid
smpp_service.ESME_RINVCMDID = 0x00000003 -- Invalid Command ID
smpp_service.ESME_RINVBNDSTS = 0x00000004 -- Incorrect BIND Status for given command
smpp_service.ESME_RALYBND = 0x00000005 -- ESME Already in Bound State
smpp_service.ESME_RINVPRTFLG = 0x00000006 -- Invalid Priority Flag
smpp_service.ESME_RINVREGDLVFLG = 0x00000007 -- Invalid Registered Delivery Flag
smpp_service.ESME_RSYSERR = 0x00000008 -- System Error
smpp_service.ESME_RINVSRCADR = 0x0000000A -- Invalid Source Address
smpp_service.ESME_RINVDSTADR = 0x0000000B -- Invalid Dest Addr
smpp_service.ESME_RINVMSGID = 0x0000000C -- Message ID is invalid
smpp_service.ESME_RBINDFAIL = 0x0000000D -- Bind Failed
smpp_service.ESME_RINVPASWD = 0x0000000E -- Invalid Password
smpp_service.ESME_RINVSYSID = 0x0000000F -- Invalid System ID
smpp_service.ESME_RCANCELFAIL = 0x00000011 -- Cancel SM Failed
smpp_service.ESME_RREPLACEFAIL = 0x00000013 -- Replace SM Failed
smpp_service.ESME_RMSGQFUL = 0x00000014 -- Message Queue Full
smpp_service.ESME_RINVSERTYP = 0x00000015 -- Invalid Service Type
smpp_service.ESME_RINVNUMDESTS = 0x00000033 -- Invalid number of destinations
smpp_service.ESME_RINVDLNAME = 0x00000034 -- Invalid Distribution List name
smpp_service.ESME_RINVDESTFLAG = 0x00000040 -- Destination flag is invalid (submit_multi)
smpp_service.ESME_RINVSUBREP = 0x00000042 -- Invalid 'submit with replace' request (i.e. submit_sm with replace_if_present_flag set)
smpp_service.ESME_RINVESMCLASS = 0x00000043 -- Invalid esm_class field data
smpp_service.ESME_RCNTSUBDL = 0x00000044 -- Cannot Submit to Distribution List
smpp_service.ESME_RSUBMITFAIL = 0x00000045 -- submit_sm or submit_multi failed
smpp_service.ESME_RINVSRCTON = 0x00000048 -- Invalid Source address TON
smpp_service.ESME_RINVSRCNPI = 0x00000049 -- Invalid Source address NPI
smpp_service.ESME_RINVDSTTON = 0x00000050 -- Invalid Destination address TON
smpp_service.ESME_RINVDSTNPI = 0x00000051 -- Invalid Destination address NPI
smpp_service.ESME_RINVSYSTYP = 0x00000053 -- Invalid system_type field
smpp_service.ESME_RINVREPFLAG = 0x00000054 -- Invalid replace_if_present flag
smpp_service.ESME_RINVNUMMSGS = 0x00000055 -- Invalid number of messages
smpp_service.ESME_RTHROTTLED = 0x00000058 -- Throttling error (ESME has exceeded allowed message limits)
smpp_service.ESME_RINVSCHED = 0x00000061 -- Invalid Scheduled Delivery Time
smpp_service.ESME_RINVEXPIRY = 0x00000062 -- Invalid message (Expiry time)
smpp_service.ESME_RINVDFTMSGID = 0x00000063 -- Predefined Message Invalid or Not Found
smpp_service.ESME_RX_T_APPN = 0x00000064 -- ESME Receiver Temporary App Error Code
smpp_service.ESME_RX_P_APPN = 0x00000065 -- ESME Receiver Permanent App Error Code
smpp_service.ESME_RX_R_APPN = 0x00000066 -- ESME Receiver Reject Message Error Code
smpp_service.ESME_RQUERYFAIL = 0x00000067 -- query_sm request failed
smpp_service.ESME_RINVOPTPARSTREAM = 0x000000C0, -- Error in the optional part of the PDU Body.
smpp_service.ESME_ROPTPARNOTALLWD = 0x000000C1 -- Optional Parameter not allowed
smpp_service.ESME_RINVPARLEN = 0x000000C2 -- Invalid Parameter Length.
smpp_service.ESME_RMISSINGOPTPARAM = 0x000000C3, -- Expected Optional Parameter missing
smpp_service.ESME_RINVOPTPARAMVAL = 0x000000C4 -- Invalid Optional Parameter Value
smpp_service.ESME_RDELIVERYFAILURE = 0x000000FE, -- Delivery Failure (data_sm_resp)
smpp_service.ESME_RUNKNOWNERR = 0x000000FF -- Unknown Error